Free PDF Download: Adobe Flash ActionScript 3.0 Programming. This manual provides a foundation for developing applications in Adobe® ActionScript® 3.0. To best understand the ideas and techniques described, you should already be familiar with general programming concepts such as data types, variables, loops, and functions. ActionScript 3.0 contains a flash.text package for all text-related APIs. The TextLineMetrics class provides detailed metrics for a line of text within a text field; it replaces the TextFormat.getTextExtent method in ActionScript 2.0.
This manual provides a foundation for developing applications in Adobe® ActionScript® 3.0. To best understand the ideas and techniques described, you should already be familiar with general programming concepts such as data types, variables, loops, and functions. You should also understand basic object-oriented programming concepts such as classes and inheritance. Prior knowledge of ActionScript 1.0 or ActionScript 2.0 is helpful but not necessary.
Here is a free download of a programming manual for Flash AS3 from Adobe.
E-Book: Flash AS3 Programming (unknown, 151 hits)
A “rough” list of this e-book’s contents:
Chapter 1: About this manual
Using this manual . . . . . . . . . 1
Accessing ActionScript documentation . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 2
ActionScript learning resources . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 3
Chapter 2: Introduction to ActionScript 3.0
About ActionScript . . . . . . . . 4
Advantages of ActionScript 3.0 . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 4
What’s new in ActionScript 3.0 . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 5
Compatibility with previous versions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 7
Chapter 3: Getting started with ActionScript
Programming fundamentals . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 9
Working with objects . . . . . 11
Common program elements . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 19
Example: Animation portfolio piece . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 21
Building applications with ActionScript . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 23
Creating your own classes 26
Example: Creating a basic application . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 29
Running subsequent examples . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 34
Chapter 4: ActionScript language and syntax
Language overview . . . . . . 37
Objects and classes . . . . . . . 38
Packages and namespaces 38
Variables . . . . . . . . . . . . . . . . . 48
Data types . . . . . . . . . . . . . . . 51
Syntax . . . . . . . . . . . . . . . . . . . 63
Operators . . . . . . . . . . . . . . . . 67
Conditionals . . . . . . . . . . . . . 73
Looping . . . . . . . . . . . . . . . . . . 75

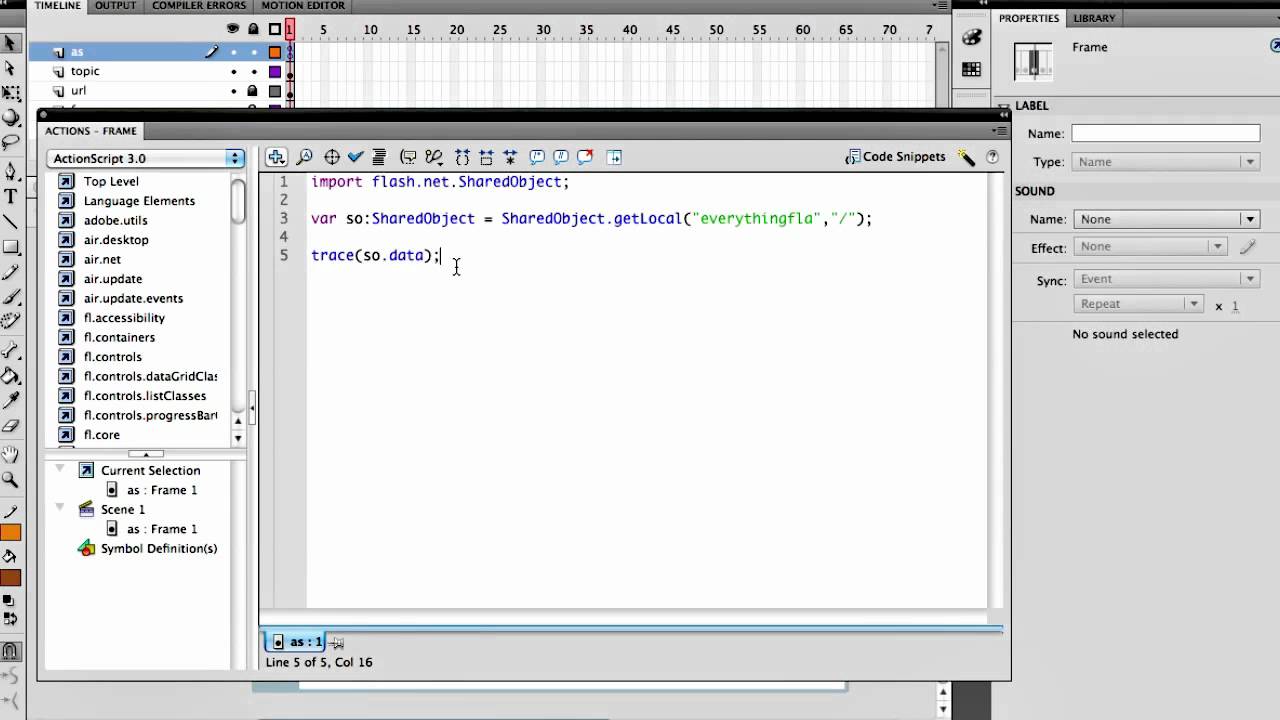
Functions . . . . . . . . . . . . . . . . 78
Chapter 5: Object-oriented programming in ActionScript
Basics of object-oriented programming . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 90
Classes . . . . . . . . . . . . . . . . . . . 91
Interfaces . . . . . . . . . . . . . . . 105
Inheritance . . . . . . . . . . . . . . 107
Advanced topics . . . . . . . . 115
Example: GeometricShapes . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 122
Chapter 6: Working with dates and times
Basics of dates and times 130
Managing calendar dates and times . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 131
PROGRAMMING ACTIONSCR IPT 3.0 FOR FLASH iv
Contents
Controlling time intervals 133
Example: Simple analog clock . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 135
Chapter 7: Working with strings
Basics of strings . . . . . . . . . 139
Creating strings . . . . . . . . . 140
The length property . . . . . 141
Working with characters in strings . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 141
Comparing strings . . . . . . . 142
Obtaining string representations of other objects . . . . . . . . . . . . . . . . . . . . . . 143
Concatenating strings . . . 143
Finding substrings and patterns in strings . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 143
Converting strings between uppercase and lowercase . . . . . . . . . . . . . . . . . . 147
Example: ASCII art . . . . . . . 148
Chapter 8: Working with arrays
Basics of arrays . . . . . . . . . . 153
Indexed arrays . . . . . . . . . . 155
Associative arrays . . . . . . . 165
Multidimensional arrays . 168
Cloning arrays . . . . . . . . . . . 169
Advanced topics . . . . . . . . 170
Example: PlayList . . . . . . . . 175
Chapter 9: Handling errors
Basics of error handling . . 179
Types of errors . . . . . . . . . . 181
Error handling in ActionScript 3.0 . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 183
Working with the debugger versions of Flash Player and AIR . . . . . . . . . . . . 184
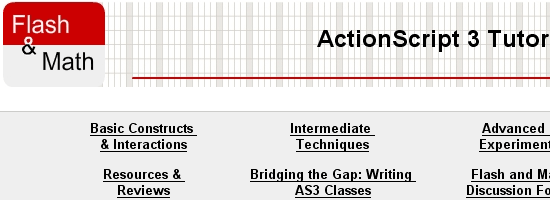
Handling synchronous errors in an application . . . . . . . . . . . . . . . . . . . . . . . . . 185
Creating custom error classes . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 189
Responding to error events and status . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 190
Comparing the Error classes . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 193
Example: CustomErrors application . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 198
Chapter 10: Using regular expressions
Basics of regular expressions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 203
Regular expression syntax . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 205
Methods for using regular expressions with strings . . . . . . . . . . . . . . . . . . . . . 217
Example: A Wiki parser . . 218
Chapter 11: Working with XML
Basics of XML . . . . . . . . . . . . 223
The E4X approach to XML processing . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 226
XML objects . . . . . . . . . . . . . 228
XMLList objects . . . . . . . . . 230
Initializing XML variables 231
Flash Actionscript 3.0
Assembling and transforming XML objects . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 232
PROGRAMMING ACTIONSCR IPT 3.0 FOR FLASH v
Contents
Traversing XML structures . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 234
Using XML namespaces . 238
XML type conversion . . . . 239
Reading external XML documents . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 240
Example: Loading RSS data from the Internet . . . . . . . . . . . . . . . . . . . . . . . . . . . 241
Chapter 12: Handling events
Basics of handling events 244
How ActionScript 3.0 event handling differs from earlier versions . . . . . . . 246
The event flow . . . . . . . . . . 248
Event objects . . . . . . . . . . . . 250
Event listeners . . . . . . . . . . . 254
Example: Alarm Clock . . . . 260
Chapter 13: Display programming
Basics of display programming . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 266
Core display classes . . . . . 270
Advantages of the display list approach . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 271
Working with display objects . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 273
Manipulating display objects . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 285
Animating objects . . . . . . . 303
Loading display content dynamically . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 304
Example: SpriteArranger . 307
Chapter 14: Using the drawing API
Basics of using the drawing API . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 314
Understanding the Graphics class . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 316
Drawing lines and curves 316
Drawing shapes using built-in methods . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 318
Creating gradient lines and fills . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 319
Using the Math class with drawing methods . . . . . . . . . . . . . . . . . . . . . . . . . . . 323
Animating with the drawing API . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 324
Example: Algorithmic Visual Generator . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 324
Advanced use of the drawing API . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 326
Drawing Paths . . . . . . . . . . 327
Defining winding rules . . 329
Using graphics data classes . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 331
About using drawTriangles() . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 333
Chapter 15: Working with geometry
Basics of geometry . . . . . . 334
Using Point objects . . . . . . 336
Using Rectangle objects . 337
Using Matrix objects . . . . . 340
Example: Applying a matrix transformation to a display object . . . . . . . . . . 342
PROGRAMMING ACTIONSCR IPT 3.0 FOR FLASH vi
Contents
Chapter 16: Filtering display objects
Basics of filtering display objects . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 346
Creating and applying filters . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 347
Available display filters . . 353
Example: Filter Workbench . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 369
Chapter 17: Working with Pixel Bender shaders
Basics of Pixel Bender shaders . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 376
Loading or embedding a shader . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 378
Accessing shader metadata . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 379
Specifying shader input and parameter values . . . . . . . . . . . . . . . . . . . . . . . . . 380
Using a shader . . . . . . . . . . 386
Chapter 18: Working with movie clips
Basics of movie clips . . . . . 398
Working with MovieClip objects . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 400
Controlling movie clip playback . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 400
Creating MovieClip objects with ActionScript . . . . . . . . . . . . . . . . . . . . . . . . . . 402
Loading an external SWF file . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 405
Example: RuntimeAssetsExplorer . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 406
Chapter 19: Working with motion tweens
Basics of Motion Tweens . 410
Copying motion tween scripts . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 411
Incorporating motion tween scripts . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 412
Describing the animation 412
Adding filters . . . . . . . . . . . . 415
Kingdom come deliverance console commands item list. Associating a motion tween with its display objects . . . . . . . . . . . . . . . . . . . . 416
Chapter 20: Working with inverse kinematics
Basics of Inverse Kinematics . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 418
Animating IK Armatures Overview . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 419
Getting information about an IK armature . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 421
Instantiating an IK Mover and Limiting Its Movement . . . . . . . . . . . . . . . . . . . 421
Moving an IK Armature . . 422
Using IK Events . . . . . . . . . . 422
Chapter 21: Working with text
Basics of working with text . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 424
Using the TextField class . 426
Using the Flash Text Engine . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 447
Chapter 22: Working with bitmaps
Basics of working with bitmaps . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 474
The Bitmap and BitmapData classes . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 476
Manipulating pixels . . . . . 478
Copying bitmap data . . . . 480
Making textures with noise functions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 481
PROGRAMMING ACTIONSCR IPT 3.0 FOR FLASH vii
Contents
Scro lling bitmaps . . . . . . . . 483
Flash Actionscript 3.0 Emulator
Taking advantage of mipmapping . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 484
Example: Animated spinning moon . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 484
Chapter 23: Working in three dimensions (3D)
Basics of 3D . . . . . . . . . . . . . 495
Understanding the 3D features of Flash Player and the AIR runtime . . . . . 496
Creating and moving 3D objects . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 498
Projecting 3D objects onto a 2D view . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 500
Example: Perspective projection . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 502
Performing complex 3D transformations . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 504
Using triangles for 3D effects . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 507
Chapter 24: Working with video
Basics of video . . . . . . . . . . 515
Understanding video formats . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 517
Understanding the Video class . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 519
Loading video files . . . . . . 520
Controlling video playback . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 520
Playing video in full-screen mode . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 522
Streaming video files . . . . 526
Understanding cue points . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 526
Writing callback methods for metadata and cue points . . . . . . . . . . . . . . . . . 527
Using cue points and metadata . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 531
Capturing camera input . 541
Sending video to a server 547
Advanced topics for FLV files . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 547
Example: Video Jukebox . 548
Chapter 25: Working with sound
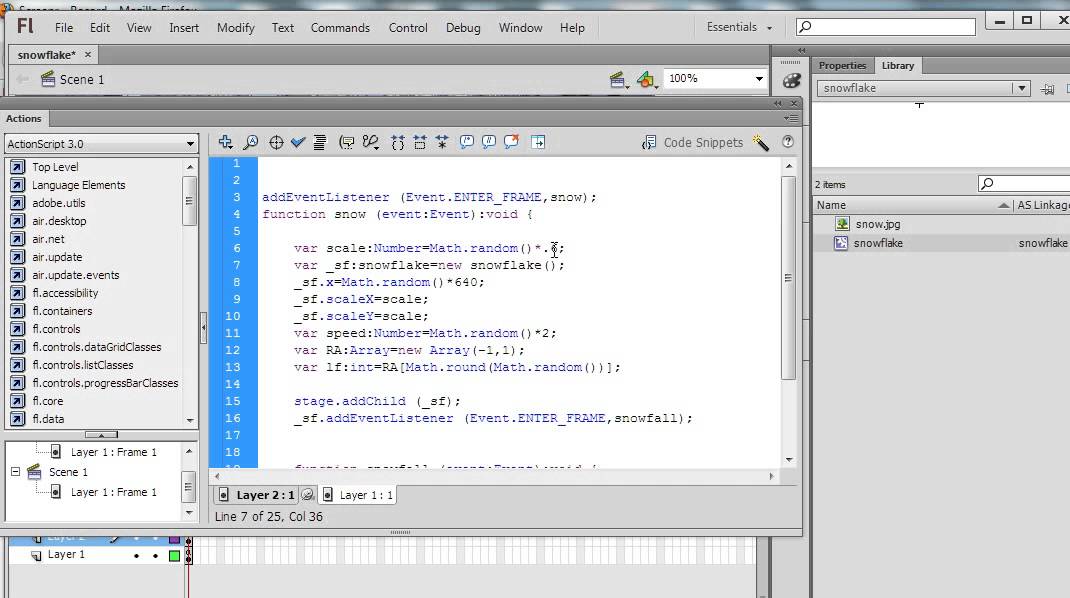
Basics of working with sound . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 554
Understanding the sound architecture . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 556
Loading external sound files . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 557
Working with embedded sounds . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 559
Working with streaming sound files . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 560
Working with dynamically generated audio . . . . . . . . . . . . . . . . . . . . . . . . . . . . 561
Playing sounds . . . . . . . . . . 563
Security considerations when loading and playing sounds . . . . . . . . . . . . . . 566
Controlling sound volume and panning . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 566
Working with sound metadata . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 568
Accessing raw sound data . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 569
Capturing sound input . . 573
Flash Actionscript 3.0 Tutorial
Example: Podcast Player . 576
Chapter 26: Capturing user input
Basics of user input . . . . . . 584
Capturing keyboard input . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 585
PROGRAMMING ACTIONSCR IPT 3.0 FOR FLASH viii
Contents
Capturi ng mouse input . . 587
Example: WordSearch . . . 591
Chapter 27: Networking and communication
Basics of networking and communication . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 595
Working with external data . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 598
Connecting to other Flash Player and AIR instances . . . . . . . . . . . . . . . . . . . . . 603
Socket connections . . . . . . 608
Storing local data . . . . . . . . 612
Working with data files . . 614
Example: Building a Telnet client . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 628
Example: Uploading and downloading files . . . . . . . . . . . . . . . . . . . . . . . . . . . . 631
Chapter 28: Client system environment
Basics of the client system environment . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 637
Using the System class . . . 639
Using the Capabilities class . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 640
Using the ApplicationDomain class . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 640
Using the IME class . . . . . . 643
Example: Detecting system capabilities . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 648
Chapter 29: Copy and paste
Copy-and-paste basics . . . 652
Reading from and writing to the system clipboard . . . . . . . . . . . . . . . . . . . . . . 652
Clipboard data formats . . 653
Chapter 30: Printing
Basics of printing . Bluestacks mac os settings. . . . . . . . 657
Printing a page . . . . . . . . . . 658
Flash Player and AIR tasks and system printing . . . . . . . . . . . . . . . . . . . . . . . . . 659
Setting size, scale, and orientation . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 661
Example: Multiple-page printing . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 663
Example: Scaling, cropping, and responding . . . . . . . . . . . . . . . . . . . . . . . . . . . 665
Chapter 31: Using the external API
Basics of using the external API . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 667
Flash Actionscript 3.0 Tutorial
External API requirements and advantages . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 669
Using the ExternalInterface class . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 670
Example: Using the external API with a web page container . . . . . . . . . . . . . 674
Example: Using the external API with an ActiveX container . . . . . . . . . . . . . 679
Chapter 32: Flash Player security
Flash Player security overview . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 685
Security sandboxes . . . . . . 686
Permission controls . . . . . 688
Restricting networking APIs . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 695
Full-screen mode security . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 697
Loading content . . . . . . . . 698
PROGRAMMING ACTIONSCR IPT 3.0 FOR FLASH ix
Contents
Cross-scripting . . . . . . . . . . 701
Accessing loaded media as data . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 703
Flash Actionscript 3.0 Download
Loading data . . . . . . . . . . . . 705
Loading embedded content from SWF files imported into a security domain . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 708
Working with legacy content . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 708
Setting LocalConnection permissions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 709
Controlling outbound URL access . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 709
Shared objects . . . . . . . . . . 711
Camera, microphone, clipboard, mouse, and keyboard access . . . . . . . . . . 712
Index . . . . . . . . . . . . . . . . . . . 713